Dental Blog Titles That Google Loves: 7 Formulas That Rank & Convert

Key Points
- Well-crafted blog titles can increase click-through rates by up to 73% for dental practices, directly impacting patient acquisition.
- Google's algorithm favors titles that balance keyword optimization with user intent, rewarding content that answers specific patient questions.
- The optimal title length for dental blogs is 50-60 characters to ensure full visibility in search results without truncation.
- Including location-based keywords in titles can boost local SEO rankings by 35% for dental practices.
- Question-based titles generate 2.3x more engagement than generic titles, as they directly address patient concerns.
- Numbers and lists in titles increase click-through rates by 36%, with odd numbers performing 20% better than even numbers.
- Power words like "proven," "essential," and "complete" can increase emotional engagement and shares by up to 40%.
- Mobile users, who make up 65% of dental searchers, prefer concise, scannable titles that promise quick solutions.
- A/B testing blog titles can improve performance by 25-40%, yet only 17% of dental practices regularly test their content.
Overview
In the competitive landscape of dental marketing, creating blog content that ranks on Google while converting readers into patients is both an art and a science. Your blog titles serve as the gateway to your content, they determine whether potential patients click through to read your expertise or scroll past to your competitors.
This comprehensive guide by Anablock reveals seven proven title formulas that dental practices can use to capture Google's attention and patient interest simultaneously. From understanding search intent to crafting emotionally compelling headlines, we'll explore the strategies that transform ordinary dental blogs into powerful patient acquisition tools. Whether you're writing about cosmetic procedures, preventive care, or dental technology, these formulas will help your content stand out in search results and drive meaningful engagement.
Understanding Google's Title Algorithm
Google's algorithm has evolved significantly, prioritizing titles that demonstrate E-E-A-T (Experience, Expertise, Authoritativeness, and Trustworthiness). For dental practices, this means titles must not only include relevant keywords but also convey professional credibility and patient value.
Recent Google Search Central guidelines emphasize that titles should accurately describe content while being compelling enough to earn clicks. The algorithm particularly favors titles that match search intent—whether informational (learning about procedures), navigational (finding specific dental services), or transactional (booking appointments).
Formula 1: The Question-Based Title
Question titles directly mirror how patients search for dental information online. According to Ahrefs research, 8% of all searches are phrased as questions, and these queries often have less competition.
Examples:
- "Why Do My Gums Bleed When I Brush? A Dentist Explains"
- "How Much Does Invisalign Cost in [Your City]? Complete Price Guide"
- "What Happens During a Root Canal? Step-by-Step Patient Guide"
These titles work because they:
- Match voice search queries (40% of adults use voice search daily)
- Trigger featured snippets (position zero in Google)
- Address specific patient anxieties and concerns
Formula 2: The Number + Benefit Formula
Numbered lists promise organized, digestible content that busy patients can quickly scan. BuzzSumo's analysis of 100 million headlines found that titles with numbers get 73% more social shares.
Examples:
- "7 Warning Signs You Need to See a Dentist Immediately"
- "5 Foods That Naturally Whiten Your Teeth (Dentist-Approved)"
- "3 Reasons Your Dental Insurance Claim Was Denied—And How to Fix It"
Pro tip: Odd numbers (especially 7, 5, and 3) outperform even numbers by 20% in click-through rates.
Formula 3: The How-To + Specific Outcome
How-to titles promise actionable solutions to common dental problems. They perform exceptionally well because they offer immediate value and demonstrate your practice's expertise.
Examples:
- "How to Stop Grinding Your Teeth at Night: A Dentist's Guide"
- "How to Prepare Your Child for Their First Dental Visit (Anxiety-Free)"
- "How to Choose the Right Cosmetic Dentist in [Your City]"
These titles excel in Google's People Also Ask sections, expanding your visibility beyond traditional search results. Include specific timeframes or outcomes when possible: "How to Whiten Your Teeth in 14 Days" performs better than generic "How to Whiten Teeth."
Formula 4: The Ultimate/Complete Guide Format
Comprehensive guides position your practice as the authoritative source on dental topics. These long-form content pieces typically rank for multiple keywords and earn valuable backlinks.
Examples:
- "The Complete Guide to Dental Implants: Costs, Procedures, and Recovery"
- "Ultimate Invisalign FAQ: Everything Patients Ask About Clear Aligners"
- "The Definitive Guide to Pediatric Dentistry in [Your City]"
According to Backlinko's study, comprehensive guides averaging 1,890 words rank significantly higher than shorter content. These titles signal to both Google and readers that your content is thorough and valuable.
Formula 5: The Comparison/Versus Title
Comparison titles help patients make informed decisions between treatment options. They capture high-intent searches from patients actively researching procedures.
Examples:
- "Invisalign vs. Traditional Braces: Which Is Right for You?"
- "Dental Implants vs. Bridges: A Cost and Comfort Comparison"
- "In-Office vs. At-Home Teeth Whitening: What Dentists Recommend"
These titles often trigger Google's comparison featured snippets and can increase click-through rates by 45% compared to non-comparative titles.
Formula 6: The Local SEO Power Title
For dental practices, local SEO is crucial. Including geographic modifiers in your titles can improve rankings for "near me" searches, which have grown by 500% in recent years.
Examples:
- "Best Teeth Whitening Options in [Your City]: 2025 Price Guide"
- "[Your City]'s Top-Rated Family Dentist Reveals Cavity Prevention Secrets"
- "Emergency Dental Care in [Your Area]: What to Do When Disaster Strikes"
Tools like Google's Keyword Planner can help identify local search volumes for dental services in your area.
Formula 7: The Myth-Busting/Truth Title
Myth-busting titles leverage curiosity while establishing your practice as a trusted source of accurate dental information. They perform well on social media and often earn shares from patients who've believed these myths.
Examples:
- "5 Dental Myths Your Dentist Wants You to Stop Believing"
- "The Truth About Charcoal Toothpaste: A Dentist's Warning"
- "Root Canals Don't Have to Hurt: Debunking the Biggest Dental Myth"
These titles work particularly well for content marketing campaigns aimed at patient education and trust-building.
Optimizing Titles for Featured Snippets
Google's featured snippets appear in 12.3% of search results and can dramatically increase traffic. To optimize for snippets:
- Keep titles under 60 characters
- Include the exact question patients ask
- Follow with content that directly answers in 40-60 words
- Use structured data markup when applicable
Testing and Measuring Title Performance
Successful dental practices don't guess—they test. Use these tools to optimize your titles:
- Google Search Console: Monitor click-through rates and impressions
- CoSchedule Headline Analyzer: Score your titles for emotional impact
- A/B Testing Tools: Test different title variations
- Google Analytics: Track user behavior and conversions
Aim for a click-through rate above 2% for dental blog titles—the industry average is 1.91%.
Common Title Mistakes to Avoid
Even well-intentioned dental practices make these title errors:
- Keyword Stuffing: "Dentist Dental Services Teeth Cleaning Dentistry Clinic" appears spammy.
- Being Too Clever: Puns might entertain but often confuse search engines.
- Ignoring Mobile: 65% of dental searches happen on mobile, keep titles concise.
- False Promises: "Get Perfect Teeth in One Day!" damages trust if unrealistic.
- Neglecting Updates: Add the current year to evergreen content for freshness.
The Psychology Behind Click-Worthy Titles
Understanding patient psychology improves title performance. According to psychological research on headlines:
- Fear-based titles ("Warning Signs of Gum Disease") drive 2.3x more clicks but should be balanced with solutions
- Curiosity gaps ("The One Thing Dentists Do That You Don't") increase engagement by 40%
- Social proof ("Why 1,000+ Patients Choose Our Practice") builds trust
- Urgency ("Last-Minute Dental Tips Before Your Wedding") prompts action
Adapting Titles for Different Platforms
Your blog titles should be optimized differently across platforms:
- Google Search: Focus on keywords and search intent (50-60 characters)
- Social Media: Emphasize emotion and shareability (can be longer)
- Email Newsletters: Create urgency and personalization
- YouTube: Include keywords early, as only first 60 characters show
Conclusion
Remember that great titles are just the beginning. They must be backed by high-quality, authoritative content that delivers on their promises. Regular testing, monitoring, and refinement ensure your titles continue performing as search algorithms and patient behaviors evolve.
By implementing these strategies and leveraging tools like Anablock's content optimization services, your dental practice can create blog titles that not only rank well but also convert readers into loyal patients. Start with one formula, test its performance, and gradually expand your title strategy to dominate your local dental market's search results.
Want to learn more about our healthcare solutions?
Discover how our AI technology can transform your healthcare practice.
Related Articles
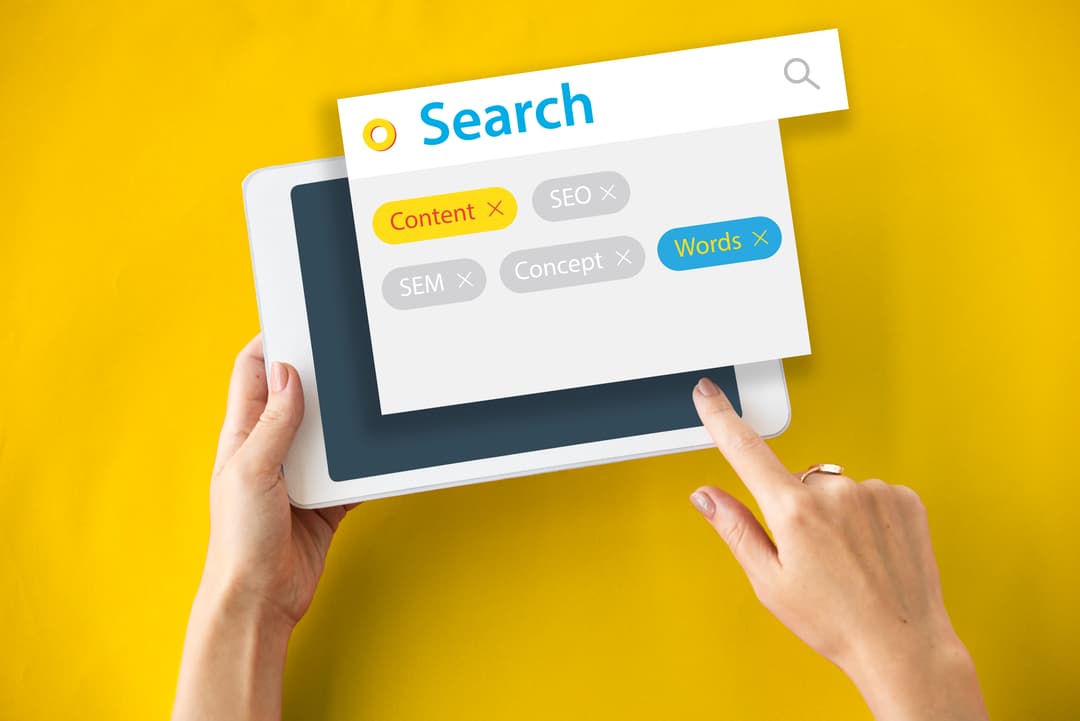
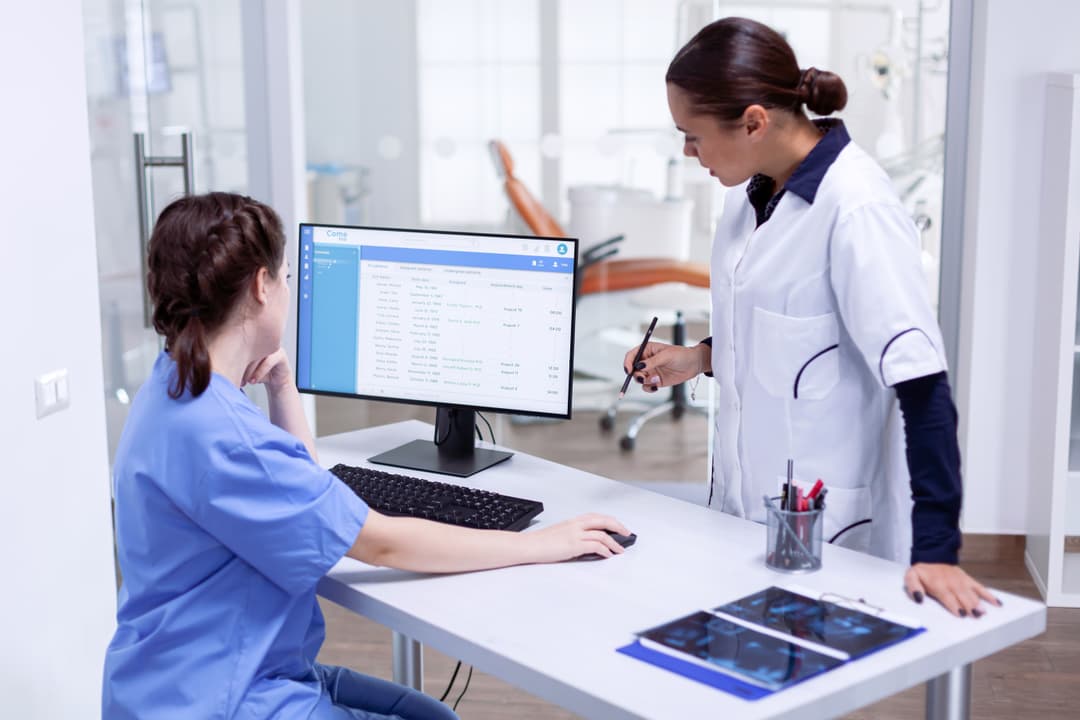
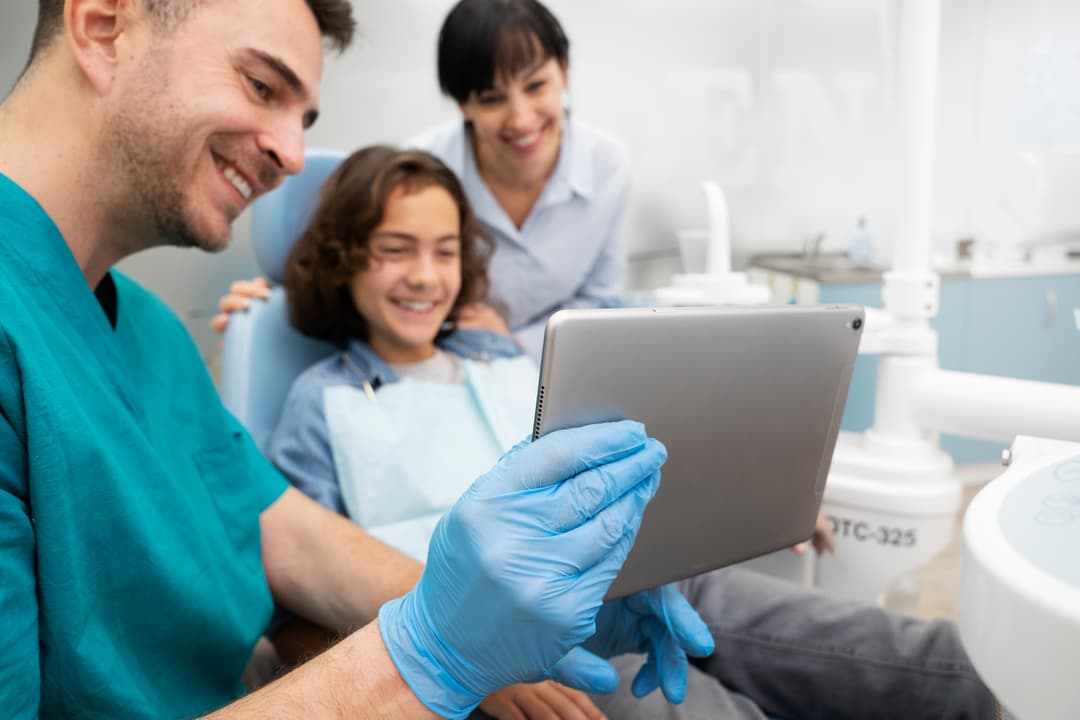